环境要求
node v12.16.3
npm 6.14.4
安装包
npm install acorn acorn-walk fs path escodegen
js代码
const acorn = require('acorn');
const walk = require('acorn-walk');
const escodegen = require('escodegen');
const fs = require('fs');
const path = require('path');
function S(e) {
for (var t = '', n = e.charCodeAt(0), i = 1; i < e.length; ++i)
t += String.fromCharCode(e.charCodeAt(i) ^ i + n & 127);
return t;
}
function recursiveDecode(node) {
if (node.type === 'Literal') {
node.value = S(node.value);
// console.log(node.value);
} else if (node.type === 'ConditionalExpression') {
recursiveDecode(node.consequent);
recursiveDecode(node.alternate);
} else {
console.log('Node type is neither Literal nor ConditionalExpression. ' + node.start);
}
}
// 这里改成你的代码位置
var inputFile = path.join(__dirname, 'ckfinder/ckfinder.min.js');
var outputFile = path.join(__dirname, 'ckfinder/ckfinder.js');
fs.readFile(inputFile, {encoding: 'utf-8'}, function (err, data) {
if (err) {
console.log(err);
return;
}
var ast = acorn.parse(data);
walk.simple(ast, {
CallExpression: function (node) {
if (node.callee.type === 'Identifier' && node.callee.name === 'S' && node.arguments.length === 1) {
var arg0 = node.arguments[0];
recursiveDecode(arg0);
if (arg0.type === 'Literal') {
} else if (arg0.type === 'ConditionalExpression') {
node.type = arg0.type;
node.test = arg0.test;
node.consequent = arg0.consequent;
node.alternate = arg0.alternate;
}
}
}
});
var code = escodegen.generate(ast);
fs.writeFile(outputFile, code, function (err) {
if (err) {
return console.log(err);
}
console.log('The file was saved!');
});
});
下面是破解的关键地方大改是
然后把S函数的return t
改为return e
function S(e) {
for (var t = '', n = e.charCodeAt(0), i = 1; i < e.length; ++i)
t += String.fromCharCode(e.charCodeAt(i) ^ i + n & 127);
return e;
}
注意点
注意一般提示都调用 dialog:info
一般出现下面代码基本都是功能限制
建议在上面代码 return true
去除页面提示
This is a demo version of CKFinder 3
function __internalInit(e) {
return {
"demoMessage": "",
"hello": "https://ckeditor.com/docs/ckfinder/ckfinder3/#!/guide/dev_translations",
"isDemo": false
}
}
//把上面的函数信息去掉
有String.fromCharCode
这块代码也是有提示的,这回是n函数。
var n = function (e) {
for (var t = '', n = 0; n < e.length; ++n)
t += String.fromCharCode(e.charCodeAt(n) ^ n - 1 & 255);
return t;
};
下面建议直接改成true,是删除文件的提示
if (!(v && y && C && m) || w) {
if (E)
return;
var n = function (e) {
for (var t = '', n = 0; n < e.length; ++n)
t += String.fromCharCode(e.charCodeAt(n) ^ n - 1 & 255);
return t;
};
setTimeout(function () {
t.setHandler('files:delete', function () {
var e = {};
e['msg'] = [
'\xA6ot',
'\x9Caollp',
'\x9Bemgwa',
'\x99imgp',
'\x96n',
'\xBBELM',
'\x92oeg-'
]['map'](n)['join'](' '), t.request('dialog:info', e);
});
}, 100), E = !0;
}
可以直接在i函数里面return "";
用PhpStorm系列软件 正则批量替换去除S函数
S\('([^']*)'\)
'$1'
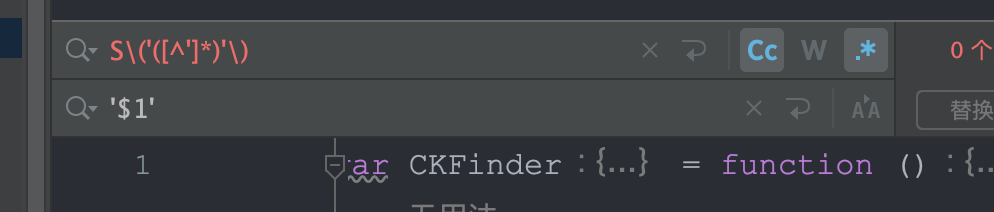